WHAT IS Browser rendering ?
This is a fundamental concept that you should really understand when learning HTML/CSS/JS and React .
Browser rendering is the process by which web browsers transform HTML, CSS, and JavaScript code into a visual representation on the screen.
This involves several steps, including parsing, constructing internal representations, applying styles, laying out elements, and painting pixels on the screen.
Steps in the Browser Rendering Process:
- Parsing HTML:
- The browser parses the HTML document into a DOM (Document Object Model) tree, representing the structure and content of the document.【将 HTML 解析成 DOM 树】
- Parsing CSS:
- CSS is parsed into a CSSOM (CSS Object Model) tree, representing the styles associated with various elements.【将 CSS 解析成 CSSOM 树】
- Combining DOM and CSSOM:
- The browser combines the DOM and CSSOM trees to create a Render Tree. The Render Tree contains the visual representation of elements to be displayed on the screen, including their styles but excluding non-visual elements like
<script>
and<meta>
tags.【合并 DOM 和 CSSOM 创建 Render tree】
- The browser combines the DOM and CSSOM trees to create a Render Tree. The Render Tree contains the visual representation of elements to be displayed on the screen, including their styles but excluding non-visual elements like
- Layout (Reflow):
- The browser calculates the layout of each Render Tree node. This step involves determining the size and position of each element on the screen. This process is called “layout” or “reflow.” 【浏览计算每个 Render Tree node 的布局】
- Painting:
- The browser converts the Render Tree into actual pixels on the screen. This step is called “painting” and involves drawing the visual representation of elements.【浏览器将 Render Tree 转换成屏幕上的像素】
- Compositing:
- For complex pages with animations, transformations, or overlapping elements, the browser may split the rendering into multiple layers. These layers are then composited together to form the final image displayed on the screen.
Detailed Breakdown:
- Parsing HTML to DOM:
- The HTML content is parsed by the browser’s HTML parser.
- A DOM tree is constructed, representing the hierarchical structure of the document.
- Parsing CSS to CSSOM:
- CSS stylesheets are parsed into the CSSOM tree.
- This tree represents all the CSS rules and their relationships.
- Creating the Render Tree:
- The browser combines the DOM and CSSOM to create the Render Tree.
- Only elements that need to be rendered (visible elements) are included in the Render Tree.
- Layout (Reflow):
- The browser calculates the exact position and size of each visible element.
- This step considers various factors such as viewport size, box model properties (margins, borders, padding), and CSS layout properties (position, float, flexbox, grid).
- Painting:
- The browser takes the Render Tree and paints the pixels onto the screen.
- This involves drawing text, colors, borders, shadows, images, and other visual aspects of elements.
- Compositing Layers:
- Modern browsers often create multiple layers to manage complex rendering scenarios.
- Layers are composited together in the correct order to produce the final image.
Example Rendering Flow:
1. HTML Code:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
<html> <head> <style> body { font-family: Arial; } .container { width: 100%; } .header { background-color: #f0f0f0; } </style> </head> <body> <div class="container"> <div class="header">Hello, World!</div> </div> </body> </html> |
2. Parsing:
- The HTML parser creates the DOM tree.
- The CSS parser creates the CSSOM tree.
3. Creating Render Tree:
- The browser combines the DOM and CSSOM trees to create the Render Tree.
1 2 3 4 5 |
RenderTree: - html - body - div.container - div.header |
4. Layout:
- The browser calculates positions and sizes for each element.
1 2 3 4 5 |
Layout: - html { width: 100%; height: 100%; } - body { width: 100%; height: 100%; font-family: Arial; } - div.container { width: 100%; } - div.header { width: 100%; background-color: #f0f0f0; } |
5. Painting:
- The browser paints the elements onto the screen, respecting their styles and layout properties.
1 2 3 |
Painting: - Draw background color of div.header. - Draw text "Hello, World!" in div.header. |
6. Compositing:
- If necessary, the browser creates multiple layers and composites them.
- This step is essential for handling z-index, transforms, and animations smoothly.
By understanding the browser rendering process, developers can optimize their web pages for better performance and faster load times, ensuring a smoother user experience.
以上就是浏览器渲染的过程。下面是扩展阅读(部分内容引用自:https://zhuanlan.zhihu.com/p/445810614)
HTML,CSS 和 JavaScript,但是浏览器是如何解析这些文件,最终将它们以像素显示在屏幕上的呢?这一过程叫做 Critical Rendering Path。Critical Rendering Path 指的是浏览器从请求 HTML,CSS,JavaScript 文件开始,到将它们最终以像素输出到屏幕上这一过程。
这个过程主要包括以下几个部分:
- 构建 DOM
- 将 HTML 解析成许多 Tokens
- 将 Tokens 解析成 Objects
- 将 Objects 组合成为一个 DOM 树
- 构建 CSSOM
- 解析 CSS 文件,并构建出一个 CSSOM 树(过程类似于 DOM 构建)
- 构建 Render
- 结合 DOM 和 CSSOM 构建出一颗 Render 树
- Layout
- 计算出元素相对于 viewport 的相对位置
- Paint
- 将 Render 树转换成像素,显示在屏幕上
值得注意的是,上面的过程并不是依次进行的,而是存在一定交叉,后面会详细解释。
在学习上面知识点之前,还得先弄明白,什么是 DOM ?
什么是 DOM ?
The HTML DOM (Document Object Model) is a programming interface for web documents. It represents the structure of a document (such as an HTML or XML document) as a tree of objects. This structure allows programs to read, manipulate, and modify the content, structure, and style of a web page dynamically. 【简单说呢,DOM 是”文档对象模型”,它将文档(如HTML或XML文档)的结构表示为对象树,然后方便程序读取或,操作,修改文档内容。】
Key Concepts of the HTML DOM:
- Tree Structure:
- The DOM represents the document as a tree of nodes. Each node in the tree is an object representing part of the document (e.g., an element, attribute, or text). (DOM将文档表示为节点树。树中的每个节点都是一个对象,表示文档的一部分(例如,元素、属性或文本)。)
- Nodes and Elements:
- Node: A single point in the DOM tree. There are different types of nodes, such as element nodes, text nodes, and attribute nodes.
- Element: A type of node that represents an HTML element. Elements can have child elements, attributes, and text content.
- Access and Manipulation:
- The DOM provides methods to access and manipulate the elements and content of a web page. For example, you can add, remove, or change elements and attributes.(DOM提供方法访问和操作页面内容和元素)
- Events:
- The DOM allows you to attach event handlers to elements. This way, you can respond to user actions like clicks, keypresses, and other events.(DOM允许你往元素上添加事件和响应用户操作)
DOM 也叫 DOM 对象,是浏览器对 HTML 文本解析后得到的,留在浏览器内存中的一个JS对象,俗称“文档对象模型”,其实dom元素就是一个浏览器生成的JavaScript对象啦。
引用自:https://zhuanlan.zhihu.com/p/545932647
我看过网上很多对dom的解释,但是我个人感觉很多都不太准确。在我看来,没那么复杂,DOM 就是一个浏览器根据网页源代码生成的一个 JavaScript 对象。
一个网页,对浏览器而言就是一个大的dom,网页中各个HTML节点,也被称为一个个小dom。从本质来看,dom是一个实实在在的东西,是一个浏览器生成的对象。
构建 DOM
构建 DOM 是必不可少的一环,浏览器从发出请求开始到得到 HTML 文件后,第一件事就是解析 HTML 成许多 Tokens,再将 Tokens 转换成 Objects,最后将 Objects 组合成一颗 DOM 树。
这个过程是一个循序渐进的过程,我们假设 HTML 文件很大,一个 RTT (Round-Trip Time) 只能得到一部分,浏览器得到这部分之后就会开始构建 DOM,并不会等到整个文档就位才开始渲染。这样做可以加快构建过程,而且由于自顶向下构建,因此后面构建的不会对前面的造成影响。
后面我们将会提到,CSSOM 则必须等到所有字节收到才开始构建。
构建 CSSOM
CSSOM (CSS Object Model),翻译过来叫做 CSS 对象模型。它的构建过程类似 DOM,当 HTML 解析中遇到 <link>
标签时,会请求对应的 CSS 文件,当 CSS 文件就位时,便开始解析它(如果遇到行内 <style>
时则直接解析),这一解析过程可以和构建 DOM 同时进行。
CSSOM 的构建必须要获得一份完整的 CSS 文件,而不像 DOM 的构建是一个循序渐进的过程。因为我们知道,CSS 文件中包含大量的样式,后面的样式会覆盖前面的样式,如果我们提前就构建 CSSOM,可能会得到错误的结果。
构建 Render Tree
这也是关键的一步,浏览器使用 DOM 和 CSSOM 构建出 Render Tree。此时不像构建 DOM 一样把所有节点构建出来,浏览器只构建需要在屏幕上显示的部分,因此像 <head>
或 <meta>
这些标签就无需构建了。同时,对于 display: none
的元素,也无需构建。
display: none
告诉浏览器这个元素无需出现在 Render Tree 中,但是 visibility: hidden
只是隐藏了这个元素,但是元素还占空间,会影响到后面的 Layout 过程,因此仍然需要出现在 Render Tree 中。
构建过程遵循以下步骤:
- 浏览器从 DOM 树开始,遍历每一个“可见”节点
- 对于每一个”可见”节点,在 CSSOM 上找到匹配的样式并应用
- 生成 Render Tree
扩展:CSS 匹配规则为何从右向左
相信大多数初学者都会认为 CSS 匹配是左向右的,其实恰恰相反。学习了 CRP,也就不难理解为什么了。
Layout
我们现在为止已经得到了所有元素的自身信息,但是还不知道它们相对于 Viewport 的位置和大小,Layout 这一过程需要计算的就是这两个信息。
根据这两个信息,Layout 输出元素的 Box Model,关于这个,我也写过一篇文章 Understand CSS Formatting Model。
目前为止,现在我们已经拿到了元素相对于 Viewport 的详细信息,所有的值都已经计算为相对 Viewport 的精确像素大小和位置,就差显示了。
Paint
浏览器将每一个节点以像素显示在屏幕上,最终我们看到页面。
这一过程需要的时间与文档大小,CSS 应用样式的多少以及复杂度,还有设备自身都有关,例如对简单的颜色进行 Paint 是简单的,但是对 box-shadow
进行 Paint 则是复杂的。
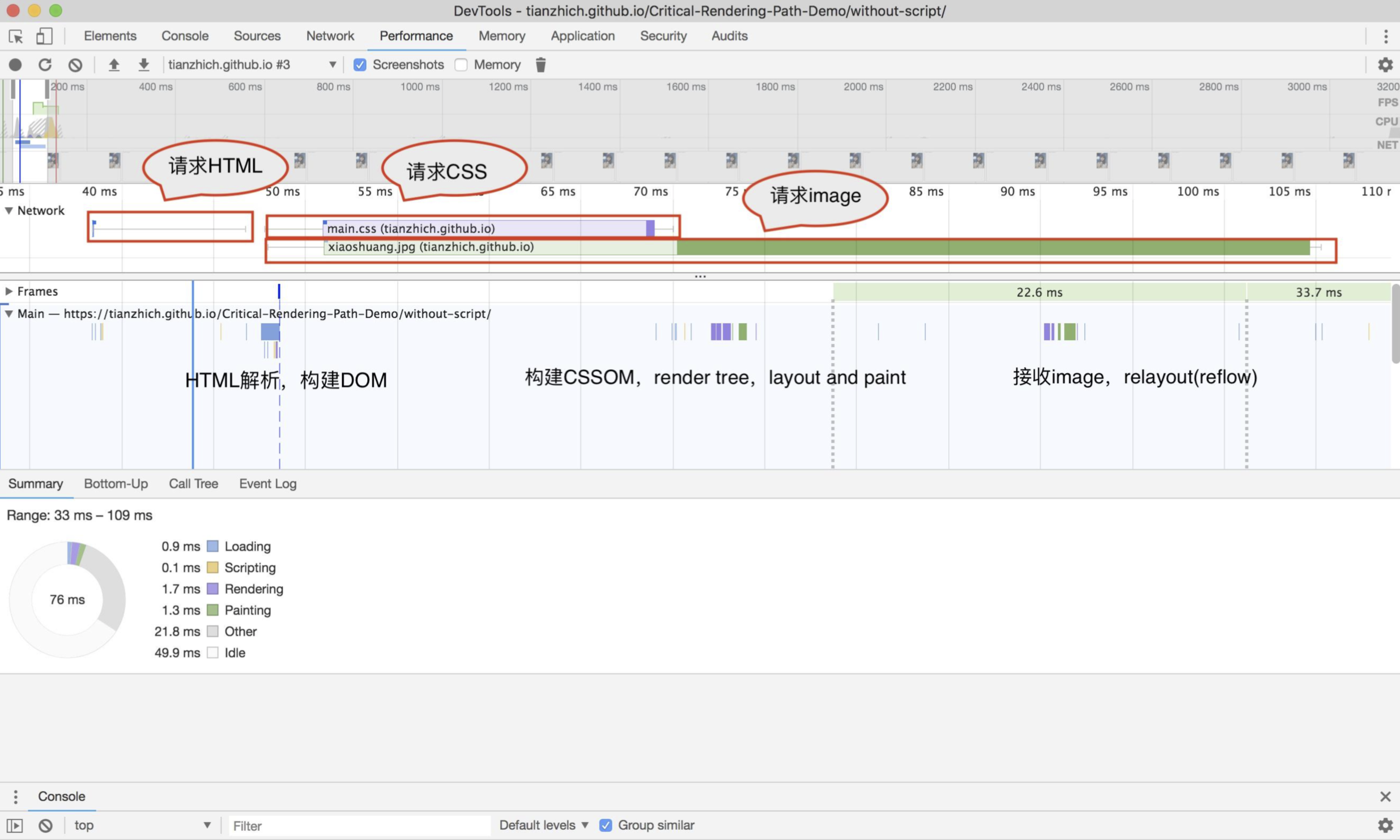
上图中,浏览器收到 HTML 文件后,便开始解析构建 DOM。(需要注意,上图显示的只是接收图片的一部分)