在 Java 中,怎么通过 http 接口传递 InputStream 流呢?
如果是同一个 JVM 方法间调用,可以直接传递 InputStream 参数,但是在 http 接口间无法直接传递 InputStream 参数,怎么办呢?
直接问 deepseek 吧:
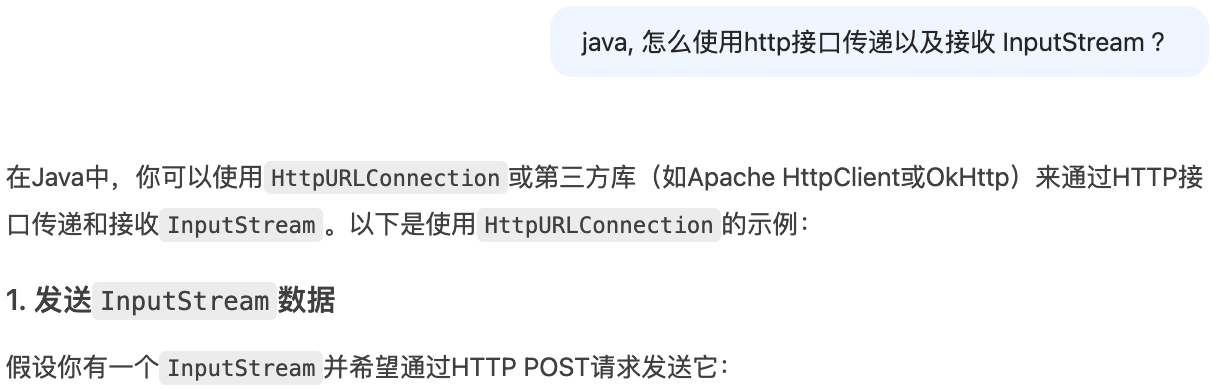
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 |
import java.io.IOException; import java.io.InputStream; import java.io.OutputStream; import java.net.HttpURLConnection; import java.net.URL; public class HttpClientExample { public static void sendInputStream(InputStream inputStream, String urlString) throws IOException { URL url = new URL(urlString); HttpURLConnection connection = (HttpURLConnection) url.openConnection(); connection.setDoOutput(true); // 允许输出 connection.setRequestMethod("POST"); connection.setRequestProperty("Content-Type", "application/octet-stream"); try (OutputStream outputStream = connection.getOutputStream()) { byte[] buffer = new byte[1024]; int bytesRead; while ((bytesRead = inputStream.read(buffer)) != -1) { outputStream.write(buffer, 0, bytesRead); } } int responseCode = connection.getResponseCode(); System.out.println("Response Code: " + responseCode); connection.disconnect(); } public static void main(String[] args) { try { InputStream inputStream = /* 获取你的InputStream */; sendInputStream(inputStream, "http://example.com/upload"); } catch (IOException e) { e.printStackTrace(); } } } |
如上所示,可以看到,发送 InputStream 流在代码中主要要做两点:
第一点:设置 connection 请求的类型
connection.setRequestProperty("Content-Type", "application/octet-stream");
第二点:获取 InputSteam 中的字节数据(该数据可以是文件流),然后写入到 connection 的 OutputStream 中:
1 2 3 4 5 6 7 |
try (OutputStream outputStream = connection.getOutputStream()) { byte[] buffer = new byte[1024]; int bytesRead; while ((bytesRead = inputStream.read(buffer)) != -1) { outputStream.write(buffer, 0, bytesRead); } } |

1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 |
import java.io.IOException; import java.io.InputStream; import java.net.HttpURLConnection; import java.net.URL; public class HttpClientExample { public static InputStream receiveInputStream(String urlString) throws IOException { URL url = new URL(urlString); HttpURLConnection connection = (HttpURLConnection) url.openConnection(); connection.setRequestMethod("GET"); int responseCode = connection.getResponseCode(); if (responseCode == HttpURLConnection.HTTP_OK) { return connection.getInputStream(); } else { throw new IOException("Failed to fetch data, response code: " + responseCode); } } public static void main(String[] args) { try { InputStream inputStream = receiveInputStream("http://example.com/download"); // 处理inputStream inputStream.close(); } catch (IOException e) { e.printStackTrace(); } } } |
上面例子中接收数据流的方法并不是我想要的,所以可以忽略上面的方法。
接收数据流就比较简单了,直接在 controller 中通过 HttpRequest 就可以拿到 InputStream 了。

1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 |
import org.apache.http.HttpEntity; import org.apache.http.client.methods.CloseableHttpResponse; import org.apache.http.client.methods.HttpPost; import org.apache.http.entity.InputStreamEntity; import org.apache.http.impl.client.CloseableHttpClient; import org.apache.http.impl.client.HttpClients; import org.apache.http.util.EntityUtils; import java.io.IOException; import java.io.InputStream; public class HttpClientExample { public static void sendInputStream(InputStream inputStream, String urlString) throws IOException { try (CloseableHttpClient httpClient = HttpClients.createDefault()) { HttpPost httpPost = new HttpPost(urlString); InputStreamEntity entity = new InputStreamEntity(inputStream); httpPost.setEntity(entity); try (CloseableHttpResponse response = httpClient.execute(httpPost)) { HttpEntity responseEntity = response.getEntity(); if (responseEntity != null) { String responseString = EntityUtils.toString(responseEntity); System.out.println("Response: " + responseString); } } } } public static void main(String[] args) { try { InputStream inputStream = /* 获取你的InputStream */; sendInputStream(inputStream, "http://example.com/upload"); } catch (IOException e) { e.printStackTrace(); } } } |
亲测好使~