What is C#?
C# is pronounced “C-Sharp”.
It is an object-oriented programming language created by Microsoft that runs on the .NET Framework.
C# has roots from the C family, and the language is close to other popular languages like C++ and Java.
The first version was released in year 2002. The latest version, C# 13, was released in November 2024.
如上所说,C#是面向对象的,C# is close to Java、C++ ,C# 是 2002 年发布,最新的 C# 版本是 C#13 发布于 2024 年。
Why Use C#?
- It is one of the most popular programming languages in the world
- It is easy to learn and simple to use
- It has huge community support
- C# is an object-oriented language which gives a clear structure to programs and allows code to be reused, lowering development costs
- As C# is close to C, C++ and Java, it makes it easy for programmers to switch to C# or vice versa
C# Output
1 2 |
Console.WriteLine("Hello World!");//会换行 Note that it will add a new line for each method Console.Write("Hello World! ");//不换行 it does not insert a new line at the end of the output |
C# Variables
Variables are containers for storing data values.
In C#, there are different types of variables (defined with different keywords), for example:
int
– stores integers (whole numbers), without decimals, such as 123 or -123double
– stores floating point numbers, with decimals, such as 19.99 or -19.99char
– stores single characters, such as ‘a’ or ‘B’. Char values are surrounded by single quotesstring
– stores text, such as “Hello World”. String values are surrounded by double quotesbool
– stores values with two states: true or false
在中文版菜鸟教程中,说 C# 中提供的基本的值类型大致可以分为以下几类:
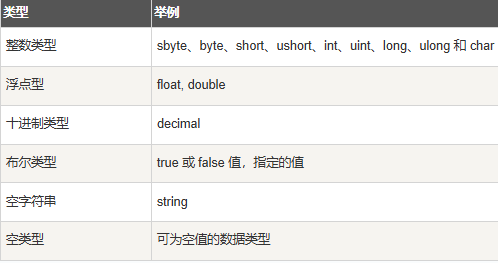
可以发现,和英文版 w3schools 中的说的类型还不太一样。
英文版 w3schools 中的例子:
1 2 3 4 5 |
int myNum = 5; double myDoubleNum = 5.99D; char myLetter = 'D'; bool myBool = true; string myText = "Hello"; |
需要注意一点:
C# 中的整型就是 int 类型,不像 Java 一样除了 int 还搞个 Integer 类型。
C# 中的类型名,都是小写的,如 int、string、char 等,不像 Java 一样是 String 、Boolean 等等。
C# Constants 常量
Constants
If you don’t want others (or yourself) to overwrite existing values, you can add the const
keyword in front of the variable type.
常量是固定值,程序执行期间不会改变。常量可以是任何基本数据类型,比如整数常量、浮点常量、字符常量或者字符串常量,还有枚举常量。
常量可以被当作常规的变量,只是它们的值在定义后不能被修改。
常量是使用 const 关键字来定义的 。
使用 const 的方法,就是将 const 关键字写在类型的前面。
This will declare the variable as “constant”, which means unchangeable and read-only:
1 2 |
const int myNum = 15; myNum = 20; // error |
Theconst
keyword is useful when you want a variable to always store the same value, so that others (or yourself) won’t mess up your code. An example that is often referred to as a constant, is PI (3.14159…).
Note: You cannot declare a constant variable without assigning the value. If you do, an error will occur: A const field requires a value to be provided.
C# Data Types
As explained in the variables chapter, a variable in C# must be a specified data type:
1 2 3 4 5 |
int myNum = 5; // Integer (whole number) double myDoubleNum = 5.99D; // Floating point number char myLetter = 'D'; // Character bool myBool = true; // Boolean string myText = "Hello"; // String |
A data type specifies the size and type of variable values.It is important to use the correct data type for the corresponding variable; to avoid errors, to save time and memory, but it will also make your code more maintainable and readable. The most common data types are:
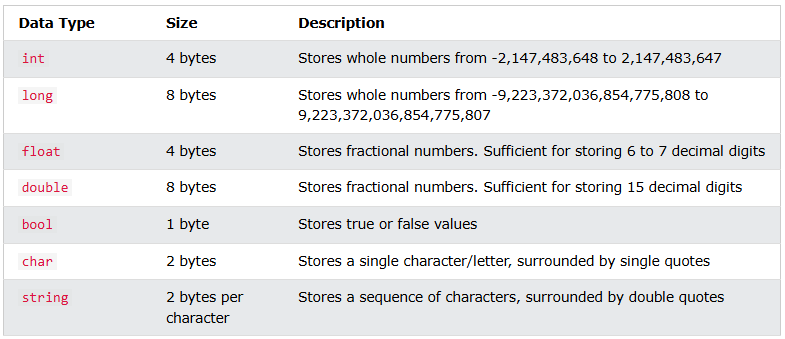
C# 菜鸟教程中是这样:
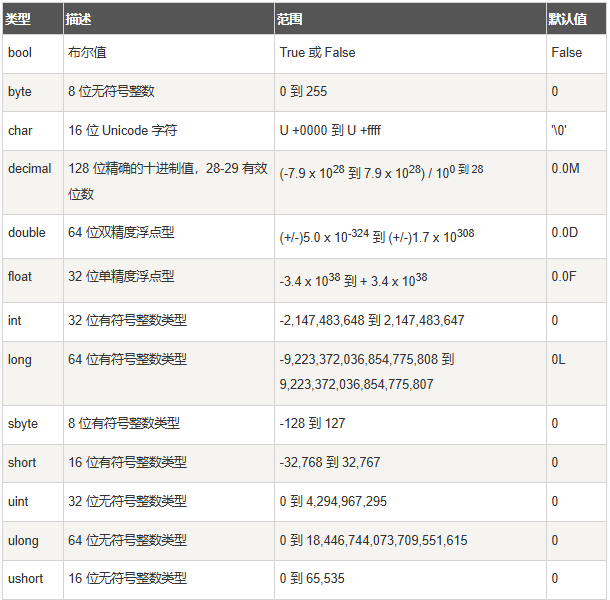
相比之下:
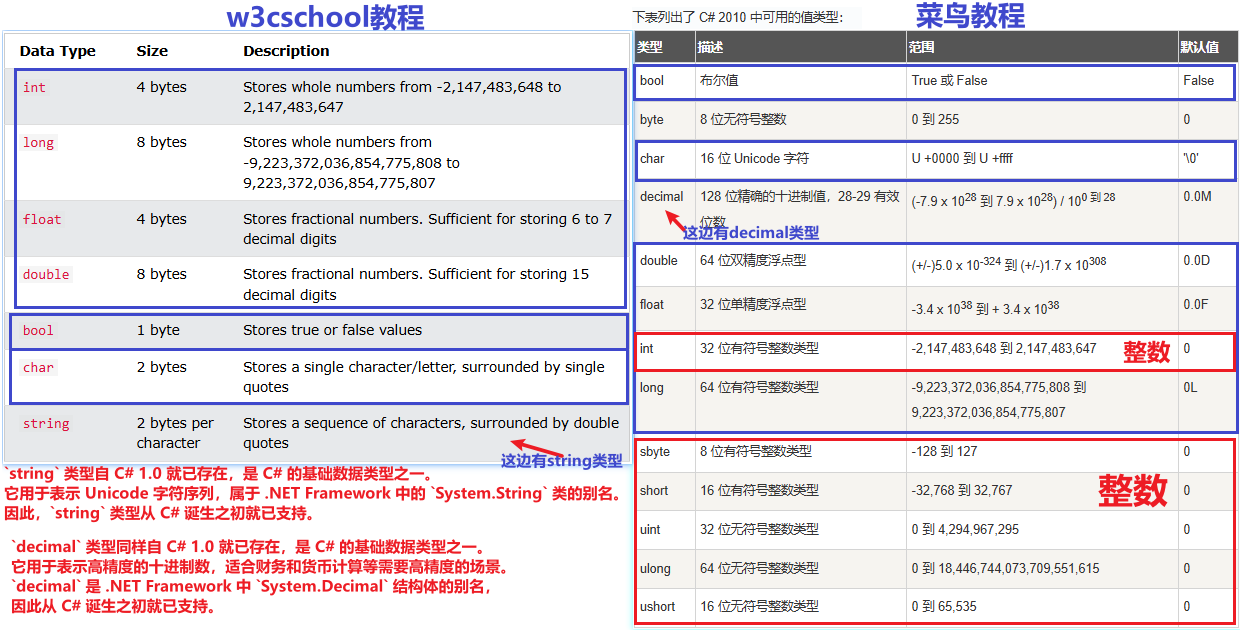
更多类型介绍:https://www.w3schools.com/cs/cs_data_types.php
C# Type Casting
Type casting is when you assign a value of one data type to another type.
In C#, there are two types of casting:
- Implicit Casting (automatically) – converting a smaller type to a larger type size
char
->int
->long
->float
->double
- Explicit Casting (manually) – converting a larger type to a smaller size type
double
->float
->long
->int
->char
Implicit Casting
Implicit casting is done automatically when passing a smaller size type to a larger size type:
1 2 3 4 5 |
int myInt = 9; double myDouble = myInt; // Automatic casting: int to double <mark style="background-color:rgba(0, 0, 0, 0)" class="has-inline-color has-vivid-purple-color"><strong>【从“small size”往“larger size”转换不要强转、不用加括号】</strong></mark> Console.WriteLine(myInt); // Outputs 9 Console.WriteLine(myDouble); // Outputs 9 |
Explicit Casting
Explicit casting must be done manually by placing the type in parentheses in front of the value:
1 2 3 4 5 |
double myDouble = 9.78; int myInt = (int) myDouble; // Manual casting: double to int<mark style="background-color:rgba(0, 0, 0, 0)" class="has-inline-color has-vivid-purple-color"><strong>【从“<mark style="background-color:rgba(0, 0, 0, 0)" class="has-inline-color has-vivid-purple-color"><strong>larger</strong></mark> size”往“<mark style="background-color:rgba(0, 0, 0, 0)" class="has-inline-color has-vivid-purple-color"><strong>small</strong></mark> size”转换需要强转、需要加括号,且可能丢失精度】</strong></mark> Console.WriteLine(myDouble); // Outputs 9.78 Console.WriteLine(myInt); // Outputs 9 |
上面都是“数字”之间的转换,如果是“数字”和“字符串”之间的转换呢?
1 2 |
int a = 1; string b = 1 + ""; // 像 Java 一样,将数字和字符串用 + 号连接即可。 |
Type Conversion Methods . C# 提供的不同类型之间转换的方法
It is also possible to convert data types explicitly by using built-in methods, such as Convert.ToBoolean
, Convert.ToDouble
, Convert.ToString
, Convert.ToInt32
(int
) and Convert.ToInt64
(long
): 【有点类似于 Java 中的 String.valueOf(..)、Integer.parseInt(…)】
1 2 3 4 5 6 7 8 |
int myInt = 10; double myDouble = 5.25; bool myBool = true; Console.WriteLine(Convert.ToString(myInt)); // <strong><mark style="background-color:rgba(0, 0, 0, 0)" class="has-inline-color has-vivid-purple-color">convert int to string</mark></strong> Console.WriteLine(Convert.ToDouble(myInt)); // <strong><mark style="background-color:rgba(0, 0, 0, 0)" class="has-inline-color has-vivid-purple-color">convert int to double</mark></strong> Console.WriteLine(Convert.ToInt32(myDouble)); // <strong><mark style="background-color:rgba(0, 0, 0, 0)" class="has-inline-color has-vivid-purple-color">convert double to int</mark></strong> Console.WriteLine(Convert.ToString(myBool)); // <strong><mark style="background-color:rgba(0, 0, 0, 0)" class="has-inline-color has-vivid-purple-color">convert bool to string</mark></strong> |
C# 中内置了很多不同类型之间的转换方法,这些方法都定义在 System.Convert 类中,使用时需要包含 System 命名空间。它们提供了一种安全的方式来执行类型转换,因为它们可以处理 null值,并且会抛出异常,如果转换不可能进行。
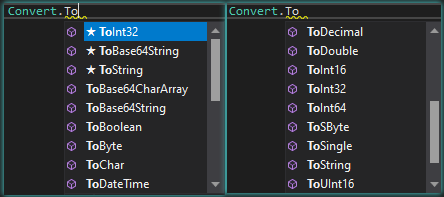
更多类型转换见:https://www.runoob.com/csharp/csharp-type-conversion.html
Arithmetic Operators
Arithmetic operators are used to perform common mathematical operations: 【运算符和 Java 基本一样】
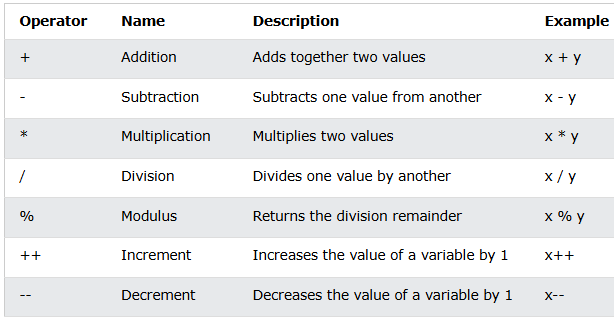
Logical Operators
As with comparison operators, you can also test for True
or False
values with logical operators.
Logical operators are used to determine the logic between variables or values:
C# For Loop
1 2 3 4 |
for (int i = 0; i < 5; i++) { Console.WriteLine(i); } |
C# Foreach Loop
1 2 3 4 5 |
string[] cars = {"Volvo", "BMW", "Ford", "Mazda"}; foreach (string i in cars) { Console.WriteLine(i); } |
C# Arrays 【和 Java 中的差不多】
1 |
string[] cars = {"Volvo", "BMW", "Ford", "Mazda"}; |
Sort an Array 【数组排序!!!】
There are many array methods available, for example Sort()
, which sorts an array alphabetically or in an ascending order:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
// Sort a string string[] cars = {"Volvo", "BMW", "Ford", "Mazda"}; Array.Sort(cars); // ██ Array.Sort(...) foreach (string i in cars) { Console.WriteLine(i); } // Sort an int int[] myNumbers = {5, 1, 8, 9}; Array.Sort(myNumbers); foreach (int i in myNumbers) { Console.WriteLine(i); } |
System.Linq Namespace ██ 使用 Linq 排序!!!
Other useful array methods, such as Min
, Max
, and Sum
, can be found in the System.Linq
namespace:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
using System; using System.Linq; namespace MyApplication { class Program { static void Main(string[] args) { int[] myNumbers = {5, 1, 8, 9}; Console.WriteLine(myNumbers.Max()); // returns the largest value Console.WriteLine(myNumbers.Min()); // returns the smallest value Console.WriteLine(myNumbers.Sum()); // returns the sum of elements } } } |
C# Multidimensional Arrays 多维数组
In the previous chapter, you learned about arrays, which is also known as single dimension arrays. These are great, and something you will use a lot while programming in C#. However, if you want to store data as a tabular form, like a table with rows and columns, you need to get familiar with multidimensional arrays.A multidimensional array is basically an array of arrays.Arrays can have any number of dimensions. The most common are two-dimensional arrays (2D).
Two-Dimensional Arrays
To create a 2D array, add each array within its own set of curly braces, and insert a comma (,
) inside the square brackets:
1 |
int[,] numbers = { {1, 4, 2}, {3, 6, 8} }; |
Good to know: The single comma [,]
specifies that the array is two-dimensional. A three-dimensional array would have two commas: int[,,]
.
numbers
is now an array with two arrays as its elements. The first array element contains three elements: 1, 4 and 2, while the second array element contains 3, 6 and 8. To visualize it, think of the array as a table with rows and columns:
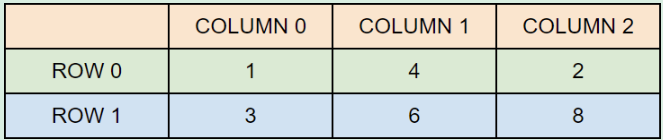
Access Elements of a 2D Array
To access an element of a two-dimensional array, you must specify two indexes: one for the array, and one for the element inside that array. Or better yet, with the table visualization in mind; one for the row and one for the column (see example below).
This statement accesses the value of the element in the first row (0) and third column (2) of the numbers
array:
1 2 |
int[,] numbers = { {1, 4, 2}, {3, 6, 8} }; Console.WriteLine(numbers[0, 2]); // Outputs 2 |
Remember that: Array indexes start with 0: [0] is the first element. [1] is the second element, etc.