Class Members
Fields and methods inside classes are often referred to as “Class Members”:
Create a Car
class with three class members: two fields and one method.
1 2 3 4 5 6 7 8 9 10 11 |
// The class class MyClass { // Class members string color = "red"; // field <strong><mark style="background-color:rgba(0, 0, 0, 0)" class="has-inline-color has-vivid-purple-color">██这里其实很关键,这里的 color 叫 Filed</mark></strong> int maxSpeed = 200; // field <strong><mark style="background-color:rgba(0, 0, 0, 0)" class="has-inline-color has-vivid-purple-color">██默认访问权限是 private</mark></strong> public void fullThrottle() // method { Console.WriteLine("The car is going as fast as it can!"); } } |
Fields
██ 这里其实很关键,注意叫 Filed ,会跟以后的 Properties 区分!!!
In the previous chapter, you learned that variables inside a class are called fields, and that you can access them by creating an object of the class, and by using the dot syntax (.
)
1 2 3 4 5 6 7 8 9 10 11 12 |
class Car { string color = "red";// <strong><mark style="background-color:rgba(0, 0, 0, 0)" class="has-inline-color has-vivid-purple-color">██默认是 private 的,只能在类里面可见!!!</mark></strong> int maxSpeed = 200; static void Main(string[] args) { Car myObj = new Car(); Console.WriteLine(myObj.color);、、 Console.WriteLine(myObj.maxSpeed); } } |
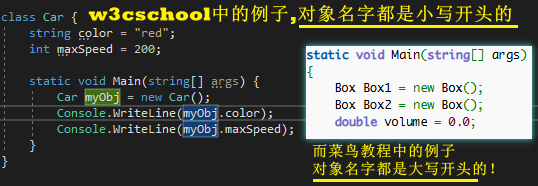
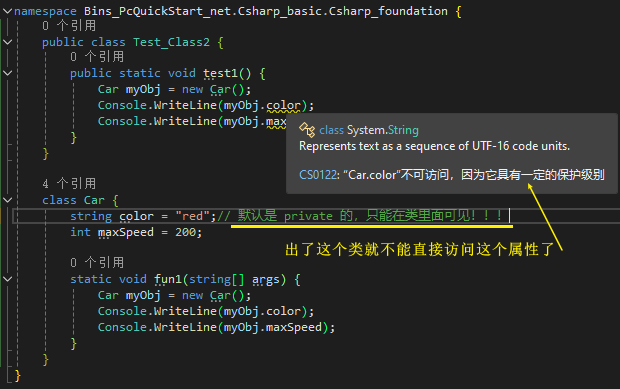
Constructors
A constructor is a special method that is used to initialize objects. The advantage of a constructor, is that it is called when an object of a class is created. It can be used to set initial values for fields.
类的 构造函数 是类的一个特殊的成员函数,当创建类的新对象时执行。
构造函数的名称与类的名称完全相同,它没有任何返回类型。
Note that the constructor name must match the class name, and it cannot have a return type (like void
or int
).
Also note that the constructor is called when the object is created.
All classes have constructors by default: if you do not create a class constructor yourself, C# creates one for you. However, then you are not able to set initial values for fields.
Constructors save time! Take a look at the last example on this page to really understand why.
默认的构造函数没有任何参数。
Access Modifiers 访问修饰符
By now, you are quite familiar with the public
keyword that appears in many of our examples:
1 |
public string color; |
The public
keyword is an access modifier, which is used to set the access level/visibility for classes, fields, methods and properties.
C# has the following access modifiers:
Modifier | Description |
---|---|
public | The code is accessible for all classes |
private | The code is only accessible within the same class |
protected | The code is accessible within the same class, or in a class that is inherited from that class. You will learn more about inheritance in a later chapter |
internal | The code is only accessible within its own assembly, but not from another assembly. You will learn more about this in a later chapter |
There’s also two combinations: protected internal
and private protected
.
C# Properties (Get and Set) ██ 重点!!!
Properties and Encapsulation
Before we start to explain properties, you should have a basic understanding of “Encapsulation“. (封装)
The meaning of Encapsulation, is to make sure that “sensitive” data is hidden from users. To achieve this, you must:
- declare fields/variables as
private
- provide
public
get
andset
methods, through properties, to access and update the value of aprivate
field
Properties
You learned from the previous chapter that private
variables can only be accessed within the same class (an outside class has no access to it). However, sometimes we need to access them – and it can be done with properties.
A property is like a combination of a variable and a method(一个属性,就像是一个“变量”和“方法”的组合 ===》这个解释很重要!!!), and it has two methods: a get
and a set
method:
1 2 3 4 5 6 7 8 9 10 |
class Person { private string name; // field public string Name // property { get { return name; } // get method set { name = value; } // set method } } |
关于“属性 properties”的理解:
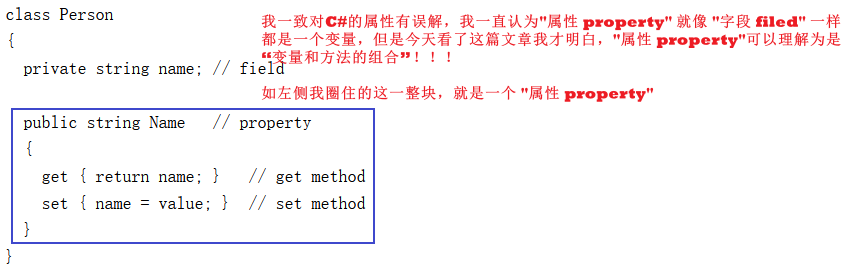
如上图所示,Name 这个”属性 property”关联到了 name 这个”字段 filed”上面。
可以看到,原先定义的专门用来 get 和 set “字段 filed” 的 public 方法没有了!!而是用 “属性 property” 来 get 和 set “字段 filed”。
The Name
property is associated with the name
field. It is a good practice to use the same name for both the property and the private field, but with an uppercase first letter【用相同的名字、但首字母大小写来区分 “属性 property” 和 “字段 filed” 是一个好的方式!】.
The get
method returns the value of the variable name
.
The set
method assigns a value
to the name
variable. The value
keyword represents the value we assign to the property.
Now we can use the Name
property to access and update the private
field of the Person
class:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 |
class Person { private string name; // field public string Name // property { get { return name; } set { name = value; } } } class Program { static void Main(string[] args) { Person myObj = new Person(); myObj.Name = "Liam"; <strong>//███操作 <mark style="background-color:rgba(0, 0, 0, 0)" class="has-inline-color has-vivid-purple-color">"属性 property"</mark> 用点 . 符号!!!</strong> Console.WriteLine(myObj.Name); } } |
Automatic Properties (Short Hand)
C# also provides a way to use short-hand / automatic properties, where you do not have to define the field for the property【你不用定义”属性field”了】, and you only have to write get;
and set;
inside the property.
The following example will produce the same result as the example above. The only difference is that there is less code:
Using automatic properties:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
class Person { public string Name // property { get; set; } } class Program { static void Main(string[] args) { Person myObj = new Person(); myObj.Name = "Liam"; Console.WriteLine(myObj.Name); } } |
更简化的定义“属性”的方式:
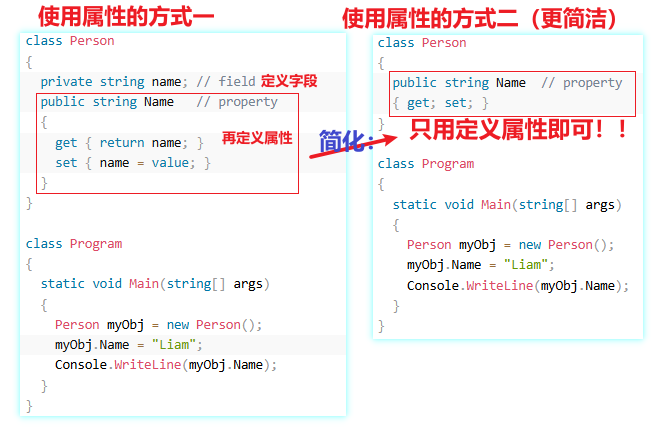
Why Encapsulation?
- Better control of class members (reduce the possibility of yourself (or others) to mess up the code)
- Fields can be made read-only (if you only use the
get
method), or write-only (if you only use theset
method) - Flexible: the programmer can change one part of the code without affecting other parts
- Increased security of data
再说属性
C# 中的属性(Property)是类和结构体中用于封装数据的成员。它们提供了一种方式来定义类成员的访问和设置规则,通常用于隐藏字段(Fields)的内部实现细节,同时提供控制数据访问的机制。
属性可以看作是对”字段的包装器”,通常由 get 和 set 访问器组成。
属性(Property)不会确定存储位置。相反,它们具有可读写或计算它们值的 访问器(accessors)。
例如,有一个名为 Student 的类,带有 age、name 和 code 的私有域。我们不能在类的范围以外直接访问这些域,但是我们可以拥有访问这些私有域的属性。
1 2 3 4 5 6 7 8 9 10 11 12 |
public class Person { private string name; public string Name { get { return name; } set { name = value; } } <strong><mark style="background-color:rgba(0, 0, 0, 0)" class="has-inline-color has-vivid-purple-color">//████上面的“属性的访问器”可以简化成这样:</mark></strong> public string Name { get; set; } } |
以上代码中,Name
属性封装了私有字段 name
。get
访问器用于获取字段值,而 set
访问器用于设置字段值。
自动实现的属性
如果你只需要一个简单的属性,C# 允许使用自动实现的属性,这样你不需要显式地定义字段!!! ██
1 2 3 4 |
public class Person { public string Name { get; set; } } |
在这种情况下,编译器会自动为 Name 属性生成一个私有的匿名字段来存储值!!!!
只读属性
如果你只需要一个只读属性,可以省略 set 访问器。
1 2 3 4 5 6 7 8 9 |
public class Person { public string Name { get; }<strong><mark style="background-color:rgba(0, 0, 0, 0)" class="has-inline-color has-vivid-purple-color">//██只读属性:只有提供 get; 访问器</mark></strong> public Person(string name) { Name = name; } } |
只写属性
类似地,如果你只需要一个只写属性,可以省略 get 访问器。
1 |
public class Person<br>{<br> private string name;<br><br> public string Name<br> {<br> set { name = value; }<strong><mark style="background-color:rgba(0, 0, 0, 0)" class="has-inline-color has-vivid-purple-color">//██只写属性:只有提供 set; 访问器</mark></strong><br> }<br>} |
自定义逻辑
你可以在 get 和 set 访问器中包含自定义的逻辑。
1 |
public class Person<br>{<br> private string name;<br><br> public string Name<br> {<br> get { return name; }<br> set <strong><mark style="background-color:rgba(0, 0, 0, 0)" class="has-inline-color has-vivid-purple-color">//██在 set; 访问器中自定义逻辑</mark></strong><br> {<br> if (string.IsNullOrWhiteSpace(value))<br> throw new ArgumentException("Name cannot be empty.");<br> name = value;<br> }<br> }<br>} |
计算属性
属性也可以是计算的,不依赖于字段。██
1 |
public class Rectangle<br>{<br> public int Width { get; set; }<strong><mark style="background-color:rgba(0, 0, 0, 0)" class="has-inline-color has-vivid-purple-color">//██属性</mark></strong><br> public int Height { get; set; }<br><br> public int Area<br> {<br> get { return Width * Height; }<br> }<br>} |
抽象属性(Abstract Properties)
抽象类可拥有抽象属性,这些属性应在派生类中被实现。下面的程序说明了这点:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 |
using System; namespace Runoob { public abstract class Person { public abstract string Name { get; set; } public abstract int Age { get; set; } } class Student : Person { // 声明自动实现的属性 public string Code { get; set; } = "N.A"; public override string Name { get; set; } = "N.A"; public override int Age { get; set; } = 0; public override string ToString() { return $"Code = {Code}, Name = {Name}, Age = {Age}"; } } class ExampleDemo { public static void Main() { // 创建一个新的 Student 对象 Student s = new Student { Code = "001", Name = "Zara", Age = 9 }; Console.WriteLine("Student Info:- {0}", s); // 增加年龄 s.Age += 1; Console.WriteLine("Student Info:- {0}", s); Console.ReadKey(); } } } |
当上面的代码被编译和执行时,它会产生下列结果:
1 2 3 |
Student Info: Code = 001, Name = Zara, Age = 9 Student Info: Code = 001, Name = Zara, Age = 10 |