C# Inheritance
Inheritance (Derived and Base Class) 派生类和基类
In C#, it is possible to inherit fields and methods from one class to another. We group the “inheritance concept” into two categories:
- Derived Class (child) – the class that inherits from another class
- Base Class (parent) – the class being inherited from
To inherit from a class, use the :
symbol 【C# 中继承使用引号 : 】
In the example below, the Car
class (child) inherits the fields and methods from the Vehicle
class (parent):
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 |
class Vehicle // base class (parent) { public string brand = "Ford"; // Vehicle field public void honk() // Vehicle method { Console.WriteLine("Tuut, tuut!"); } } class Car : Vehicle // <strong><mark style="background-color:rgba(0, 0, 0, 0)" class="has-inline-color has-vivid-purple-color">derived class (child) 派生类</mark></strong> { public string modelName = "Mustang"; // Car field } class Program { static void Main(string[] args) { // Create a myCar object Car myCar = new Car(); // Call the honk() method (From the Vehicle class) on the myCar object myCar.honk(); <strong>//█调用父类中的方法</strong> // Display the value of the brand field (from the Vehicle class) and the value of the modelName from the Car class Console.WriteLine(myCar.brand + " " + myCar.modelName); } } |
Why And When To Use “Inheritance”?
– It is useful for code reusability: reuse fields and methods of an existing class when you create a new class.
Tip: Also take a look at the next chapter, Polymorphism, which uses inherited methods to perform different tasks.
The sealed Keyword █ sealed 关键字
如果你不想让别的类继承 Vehicle 类,则可以用 sealed 关键字修饰 Vehicle 类。
If you don’t want other classes to inherit from a class, use the sealed
keyword:
If you try to access a sealed
class, C# will generate an error:
1 2 3 4 5 6 7 8 9 |
sealed class Vehicle { ... } class Car : Vehicle { ... } |
C# Polymorphism 【/ˌpɑlɪ’mɔrfɪzm/】、virtual、override
Polymorphism and Overriding Methods
Polymorphism means “many forms”, and it occurs when we have many classes that are related to each other by inheritance.
Like we specified in the previous chapter; Inheritance lets us inherit fields and methods from another class. Polymorphism uses those methods to perform different tasks. This allows us to perform a single action in different ways.
For example, think of a base class called Animal
that has a method called animalSound()
. Derived classes of Animals could be Pigs, Cats, Dogs, Birds – And they also have their own implementation of an animal sound (the pig oinks, and the cat meows, etc.):
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 |
class Animal // Base class (parent) { public void animalSound() { Console.WriteLine("The animal makes a sound"); } } class Pig : Animal // Derived class (child) { public void animalSound() { Console.WriteLine("The pig says: wee wee"); } } class Dog : Animal // Derived class (child) { public void animalSound() { Console.WriteLine("The dog says: bow wow"); } } |
Remember from the Inheritance chapter that we use the :
symbol to inherit from a class.
Now we can create Pig
and Dog
objects and call the animalSound()
method on both of them:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 |
class Animal // Base class (parent) { public void animalSound() { Console.WriteLine("The animal makes a sound"); } } class Pig : Animal // Derived class (child) { public void animalSound() { Console.WriteLine("The pig says: wee wee"); } } class Dog : Animal // Derived class (child) { public void animalSound() { Console.WriteLine("The dog says: bow wow"); } } class Program { static void Main(string[] args) { Animal myAnimal = new Animal(); // Create a Animal object Animal myPig = new Pig(); // Create a Pig object Animal myDog = new Dog(); // Create a Dog object myAnimal.animalSound(); myPig.animalSound(); myDog.animalSound(); } } |
The output will be: 【████ C# 中为什么这样输出呢?因为你创建了不同的子类对象,但是是用父类的引用接收的!!所以会优先调用父引用中的方法!】
1 2 3 |
The animal makes a sound The animal makes a sound The animal makes a sound |
和上面一模一样的 Java 代码,能自动实现多态(如下),不需要加 virtual、override 关键字:
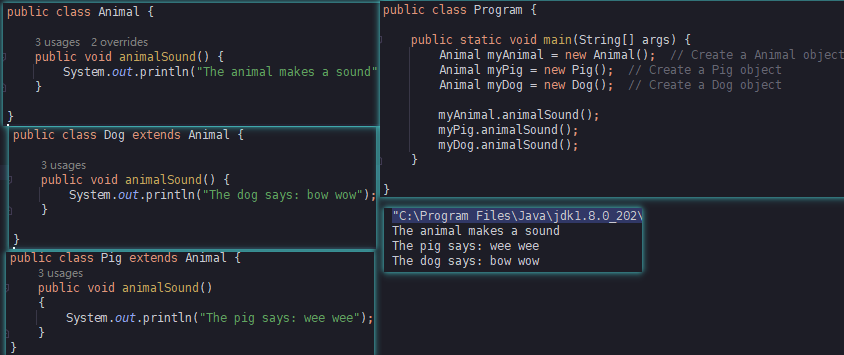
至于为什么 C# 中的多态这样实现,见最下方分析。
C# 中如果想达到想上面 Java 中的效果,得使用 virtual、override 关键字!!!
Not The Output I Was Looking For
The output from the example above was probably not what you expected. That is because the base class method overrides the derived class method, when they share the same name.
However, C# provides an option to override the base class method, by adding the virtual
keyword to the method inside the base class, and by using the override
keyword for each derived class methods:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 |
class Animal // Base class (parent) { public virtual void animalSound() <strong><mark style="background-color:rgba(0, 0, 0, 0)" class="has-inline-color has-vivid-purple-color">//██ 父类中使用 virtual 修饰方法!!!</mark></strong> { Console.WriteLine("The animal makes a sound"); } } class Pig : Animal // Derived class (child) { public override void animalSound() <strong><mark style="background-color:rgba(0, 0, 0, 0)" class="has-inline-color has-vivid-purple-color">//██ 子类中使用 override 修饰方法!!!</mark></strong> { Console.WriteLine("The pig says: wee wee"); } } class Dog : Animal // Derived class (child) { public override void animalSound() { Console.WriteLine("The dog says: bow wow"); } } class Program { static void Main(string[] args) { Animal myAnimal = new Animal(); // Create a Animal object Animal myPig = new Pig(); // Create a Pig object Animal myDog = new Dog(); // Create a Dog object myAnimal.animalSound(); myPig.animalSound(); myDog.animalSound(); } } |
The output will be:
1 2 3 |
The animal makes a sound The pig says: wee wee The dog says: bow wow |
Why And When To Use “Inheritance” and “Polymorphism”?
– It is useful for code reusability: reuse fields and methods of an existing class when you create a new class.
从上面可以看出,C# 中的多态和 Java 中的多态是有区别的,为什么 C# 中的多态这样实现呢?
C# 中为什么要加入 virtual、override 关键字?而不是像 Java 那样实现多态?
在 C# 中引入 virtual
和 override
关键字是为了更明确地控制方法的多态行为,同时提供更高的灵活性和安全性。这与 Java 的设计哲学有所不同,以下是具体原因:
1. 明确多态行为的意图
- 在 C# 中,
virtual
关键字用于标记一个方法可以被派生类重写,而override
关键字用于明确表示派生类正在重写基类的虚方法。 - 这种设计使得代码的意图更加清晰:开发者可以清楚地知道哪些方法是设计为可重写的,哪些方法是明确被重写的。
- 相比之下,Java 默认所有非静态方法都是虚方法(除非用
final
修饰),这种设计虽然简化了语法,但可能导致开发者无意中重写方法,增加了代码的不可控性。
2. 避免意外的重写
- C# 的设计要求显式使用
virtual
和override
,这样可以避免派生类无意中重写基类方法的情况。 - 在 Java 中,如果基类的方法没有被标记为
final
,派生类可以直接重写它,这可能会导致意外的行为,尤其是在大型项目中。 - C# 的这种设计提高了代码的可维护性和安全性。
3. 支持方法隐藏(Method Hiding)
- C# 提供了
new
关键字,用于隐藏基类的方法(而不是重写)。这种行为与 Java 不同,允许开发者在派生类中定义与基类同名的方法,而不会影响基类的行为。 - 这种设计在某些场景下非常有用,例如当派生类需要实现与基类同名但行为不同的方法时。
4. 性能优化
- C# 的设计允许编译器更好地优化代码。由于虚方法的调用需要通过虚表(vtable)进行动态分派,性能上会有一定的开销。
- 通过显式标记
virtual
和override
,C# 可以让开发者更清楚地知道哪些方法是虚方法,从而在性能敏感的场景中避免不必要的虚方法调用。
5. 与 C++ 的设计哲学一致
- C# 的设计受到了 C++ 的影响。在 C++ 中,虚方法也需要显式标记为
virtual
,而派生类需要显式重写。 - 这种设计哲学强调显式性和可控性,与 Java 的隐式多态设计形成对比。
6. Java 的设计哲学
- Java 的设计更倾向于简化语法和降低学习门槛。默认情况下,所有非静态方法都是虚方法,除非用
final
修饰。 - 这种设计虽然简化了代码,但也可能导致一些问题,例如:
- 开发者可能无意中重写基类方法。
- 缺乏对方法隐藏的显式支持。
总结
C# 引入 virtual
和 override
关键字的主要目的是为了提供更明确、更安全的多态机制。这种设计强调显式性和可控性,避免了意外的重写行为,同时支持方法隐藏和性能优化。相比之下,Java 的设计更注重简洁性,但可能在大型项目中带来一些不可控的风险。两种语言的设计哲学各有优劣,适用于不同的开发场景。