C# Generics
Introduction to the C# generics 【泛型方法】
C# generics allow you to write code that works with more than one type. By using generics, you can write code with placeholders for types and then provide the actual types when using the code.
Generics introduces the concept of type parameters to .NET. Generics make it possible to design classes and methods that(指”classes and methods“) defer the specification of one or more type parameters until you use the class or method in your code. 【泛型引入了类型参数的概念。泛型使设计类和方法成为可能,这些类和方法可以推迟一个或多个类型参数的规范,直到您在代码中使用该类或方法。】
Suppose you need to write a method that swaps the values of two integer variables. To do that, you can define a method called SwapInt()
that accepts two integer parameters like this:
1 2 3 4 5 6 |
static void SwapInt(ref int a, ref int b) { int temp = a; a = b; b = temp; } |
Later, you want to swap the values of two string variables. In this case, you need to define a new method that swaps the strings:
1 2 3 4 5 6 |
static void SwapString(ref string a, ref string b) { string temp = a; a = b; b = temp; } |
Both SwapInt()
and SwapString()
methods have the same logic except for the types of the variables that they deal with.
Imagine that you need to swap values of two variables of a new type e.g., float, you need to define a new method for each new type. As the result, you’ll have lots of Swap*
methods with the same logic.
To resolve this, you can create a generic method that swaps values of variables of any type like this:
1 2 3 4 5 6 |
static void Swap<T>(ref T a, ref T b) { T temp = a; a = b; b = temp; } |
In this Swap()
method, instead of using a specific type, we use the placeholder T for the type.
When using the Swap()
method, you can specify the specific type. For example:
int x = 10, y = 20; Swap<int>(ref x, ref y);
In this example, we specify the int
type inside the angle brackets (<>
) that follows the method name. Since the types of x
and y
are int
, you can make the method call short like this:
1 2 3 |
int x = 10, y = 20; Swap(ref x, ref y); |
Summary
- C# generics allow you to write general-purpose code that uses the same type in multiple places without knowing that type upfront.
- Use C# generics to write reusable, type-neutral code.
更多泛型的使用
C# allows you to use generics with the following:
- Classes
- Methods
- Interfaces
- Delegates
- Structs
In practice, you’ll find that generics are extensively used in the collection types like List<T>
, Stack<T>
, Queue<T>
, etc. These are called generic collection types.
泛型类
Introduction to the objects and classes
Objects are one of the essential concepts in object-oriented programming. Objects have states and behaviors:
- States represent the data that the object holds at a particular point in time.(状态表示对象在特定时间点保存的数据。)
- Behaviors represent the actions that the object can perform to manipulate its states.(行为表示对象可以执行来操纵其状态的动作。)
C# uses class-based object-oriented programming. Before creating objects, you need to define a class. A class is a blueprint for creating objects.
██ C# 中”泛型类”的语法和 Java 中一样:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 |
public class MyGenericArray<T> { private T[] array; public MyGenericArray(int size) { array = new T[size + 1]; } public T getItem(int index) { return array[index]; } public void setItem(int index, T value) { array[index] = value; } } <strong>// 创建泛型类(声明一个整型数组)</strong> MyGenericArray<int> intArray = new MyGenericArray<int>(5); |
泛型(Generic)方法
C# 中泛型方法的声明和 Java 中的语法不太一样:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 |
class Program { static void Swap<T>(ref T lhs, ref T rhs) { T temp; temp = lhs; lhs = rhs; rhs = temp; } static void Main(string[] args) { int a, b; char c, d; a = 10; b = 20; c = 'I'; d = 'V'; // 在交换之前显示值 Console.WriteLine("Int values before calling swap:"); Console.WriteLine("a = {0}, b = {1}", a, b); Console.WriteLine("Char values before calling swap:"); Console.WriteLine("c = {0}, d = {1}", c, d); // 调用 swap Swap<int>(ref a, ref b); Swap<char>(ref c, ref d); // 在交换之后显示值 Console.WriteLine("Int values after calling swap:"); Console.WriteLine("a = {0}, b = {1}", a, b); Console.WriteLine("Char values after calling swap:"); Console.WriteLine("c = {0}, d = {1}", c, d); Console.ReadKey(); } } |
C# 中的方法声明泛型,和 Java 中的方法声明泛型时,语法区别如下:
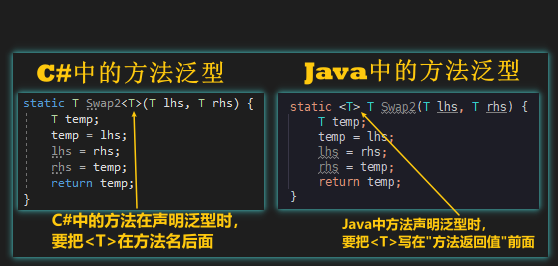
C# Anonymous Methods 匿名方法
匿名方法(Anonymous methods) 提供了一种传递代码块作为委托参数的技术。
在匿名方法中您不需要指定返回类型,它是从方法主体内的 return 语句推断的。
Lambda 表达式
Lambda 表达式是一个简洁的语法,用于创建匿名函数。它们通常用于 LINQ 查询和委托。
语法:
1 2 3 |
(parameters) => expression // 或 (parameters) => { statement; } |
示例:
1 2 3 4 5 6 7 8 9 10 11 |
// 示例:使用 Lambda 表达式定义一个委托 Func<int, int, int> add = (a, b) => a + b; Console.WriteLine(add(2, 3)); // 输出 5 // 示例:使用 Lambda 表达式过滤数组中的元素 int[] numbers = { 1, 2, 3, 4, 5 }; var evenNumbers = numbers.Where(n => n % 2 == 0); foreach (var num in evenNumbers) { Console.WriteLine(num); // 输出 2 4 } |
匿名方法
匿名方法是通过使用 delegate 关键字创建委托实例来声明的【这句话说的不严谨啊】。
语法
1 |
delegate(parameters) { statement; } |
例如:
1 2 3 4 5 6 |
delegate void NumberChanger(int n); ... NumberChanger nc = delegate(int x) { Console.WriteLine("Anonymous Method: {0}", x); }; |
代码块 Console.WriteLine(“Anonymous Method: {0}”, x); 是匿名方法的主体。
委托可以通过匿名方法调用,也可以通过命名方法调用,即,通过向委托对象传递方法参数。
注意: 匿名方法的主体后面需要一个 ; 。
例如:
1 |
nc(10); |
1 2 3 4 5 6 7 8 9 10 11 12 13 |
// 示例:使用匿名方法定义一个委托 Func<int, int, int> multiply = delegate (int a, int b) { return a * b; }; Console.WriteLine(multiply(2, 3)); // 输出 6 // 示例:使用匿名方法作为事件处理程序 Button button = new Button(); button.Click += delegate (object sender, EventArgs e) { Console.WriteLine("Button clicked!"); }; |
下面的实例演示了匿名方法的概念:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 |
using System; delegate void NumberChanger(int n); namespace DelegateAppl { class TestDelegate { static int num = 10; public static void AddNum(int p) { num += p; Console.WriteLine("Named Method: {0}", num); } public static void MultNum(int q) { num *= q; Console.WriteLine("Named Method: {0}", num); } static void Main(string[] args) { // 使用匿名方法创建委托实例 NumberChanger nc = delegate(int x) { Console.WriteLine("Anonymous Method: {0}", x); }; // 使用匿名方法调用委托 nc(10); // 使用命名方法实例化委托 nc = new NumberChanger(AddNum); // 使用命名方法调用委托 nc(5); // 使用另一个命名方法实例化委托 nc = new NumberChanger(MultNum); // 使用命名方法调用委托 nc(2); Console.ReadKey(); } } } |
当上面的代码被编译和执行时,它会产生下列结果:
1 2 3 |
Anonymous Method: 10 Named Method: 15 Named Method: 30 |
在 C# 2.0 及更高版本中,引入了 lambda 表达式,它是一种更简洁的语法形式,用于编写匿名方法。
使用 lambda 表达式:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 |
using System; delegate void NumberChanger(int n); namespace DelegateAppl { class TestDelegate { static int num = 10; public static void AddNum(int p) { num += p; Console.WriteLine("Named Method: {0}", num); } public static void MultNum(int q) { num *= q; Console.WriteLine("Named Method: {0}", num); } static void Main(string[] args) { // 使用 lambda 表达式创建委托实例 NumberChanger nc = x => Console.WriteLine($"Lambda Expression: {x}"); // 使用 lambda 表达式调用委托 nc(10); // 使用命名方法实例化委托 nc = new NumberChanger(AddNum); // 使用命名方法调用委托 nc(5); // 使用另一个命名方法实例化委托 nc = new NumberChanger(MultNum); // 使用命名方法调用委托 nc(2); Console.ReadKey(); } } } |
感觉实例中注释有点让人绕 ,个人理解是委托去调用方法。
举个例子,有一个委托中带有一个方法A,之后将委托变量传递给一个方法B(或函数) ,然后函数B在执行的时候,会通过委托去调用方法A 。但注释中让我理解为是方法A去调用的委托,这个有点费解,如果这边理不清,事件那边可能会有点乱。
还有本章中匿名方法的定义是没有名称只有方法主体, 所以通过匿名方法去调用委托也有点牵强,总不能是方法体去调用委托吧,让委托干啥,委托应该有点懵逼。
关于匿名方法这块儿我的理解是:由于匿名方法没有方法签名,只有方法体,所以无法使用命名方法类似的 方法名(); 去调用,所以只能将由委托变量去调用它,换言之,匿名方法将自己唯一拥有的方法主体交给委托,让委托代理执行。
以上仅是个人理解,但感觉这么理解比较容易接受,刚入门学习,如理解上有误还请指教
https://www.csharptutorial.net/csharp-tutorial/csharp-anonymous-methods/
When assigning a method to a delegate, you define a method, either instance method or static method, and then assign it to the delegate. For example:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
class Program { delegate int Transfomer(int x); static int Square(int x) { return x * x; } public static void Main(string[] args) { Transfomer transform = Square; Console.WriteLine(transform(10)); // 100 } } |
In this example:
First, declare a delegate called Transformer
that accepts an integer and returns an integer:
1 |
delegate int Transfomer(int x); |
Second, define a static method called Square()
with the same signature as the Transformer
delegate:
1 2 3 4 |
static int Square(int x) { return x * x; } |
Third, assign the Square
method to the Transformer
delegate and invoke it inside the Main()
method:
1 2 |
Transfomer transform = Square; Console.WriteLine(transform(10)); // 100 |
Sometimes, defining a new method is not necessary because you only use it once. It creates unwanted overhead.
C# allows you to create a delegate and immediately assign a code block to it. This code block can be either an anonymous method or a lambda expression.
An anonymous method uses the delegate
keyword followed by an optional parameter declaration and method body. For example:
1 2 3 4 5 6 7 8 9 10 11 |
class Program { delegate int Transfomer(int x); public static void Main(string[] args) { Transfomer transform = delegate (int x) { return x * x; }; Console.WriteLine(transform(10)); // 100 } } |
In this example, we don’t define the static method Square
. Instead, we assign an anonymous method to the transform
delegate.
The following statement defines an anonymous method and assigns it to the Transformer
delegate:
1 |
delegate (int x) { return x * x; } |
Anonymous methods can access the outer variables. For example:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
class Program { delegate decimal Pricing(decimal price); public static void Main(string[] args) { decimal tax = 0.08m; decimal price = 100m; Pricing calculateNet = delegate(decimal price) { return price * (1 + tax); }; Console.WriteLine(calculateNet(price)); } } |
输出:
1 |
108 |
In this example, the anonymous method accesses the tax
variable from the Main()
method.
Starting from C# 3, you can use lambda expressions instead of anonymous methods, which have more concise syntax.
Summary
- Anonymous methods are methods without names.
- Use the
delegate
keyword to define an anonymous method.